Note
Go to the end to download the full example code.
6.7-kW SyRM, saturated#
This example simulates sensorless stator-flux-vector control of a saturated 6.7-kW synchronous reluctance motor drive.
import numpy as np
import motulator.drive.control.sm as control
from motulator.drive import model, utils
Compute base values based on the nominal values (just for figures).
nom = utils.NominalValues(U=370, I=15.5, f=105.8, P=6.7e3, tau=20.1)
base = utils.BaseValues.from_nominal(nom, n_p=2)
Configure the system model.
curr_map = utils.SaturationModelSyRM(
a_d0=17.4, a_dd=373, S=5, a_q0=52.1, a_qq=658, T=1, a_dq=1120, U=1, V=0
)
par = model.SaturatedSynchronousMachinePars(
n_p=2, R_s=0.54, i_s_dq_fcn=curr_map, kind="rel"
)
machine = model.SynchronousMachine(par)
mechanics = model.MechanicalSystem(J=0.015)
converter = model.VoltageSourceConverter(u_dc=540)
mdl = model.Drive(machine, mechanics, converter)
Configure the control system, including the saturation model.
# Compute a rectilinear flux map
psi_d_range = np.linspace(-1.5 * base.psi, 1.5 * base.psi, 256)
psi_q_range = np.linspace(-0.5 * base.psi, 0.5 * base.psi, 256)
flux_map = curr_map.as_magnetic_model(psi_d_range, psi_q_range).invert()
# Plot the flux map
utils.plot_maps(flux_map, base)
# Parameter estimates
est_par = control.SaturatedSynchronousMachinePars(
n_p=2, R_s=0.54, i_s_dq_fcn=curr_map, psi_s_dq_fcn=flux_map, kind="rel"
)
# Configure the controller
cfg = control.FluxVectorControllerCfg(i_s_max=2 * base.i, psi_s_min=0.5 * base.psi)
vector_ctrl = control.FluxVectorController(est_par, cfg, sensorless=True)
speed_ctrl = control.SpeedController(J=0.015, alpha_s=2 * np.pi * 4)
ctrl = control.VectorControlSystem(vector_ctrl, speed_ctrl)
Plot control characteristics.
Set the speed reference and the external load torque.
Create the simulation object, simulate, and plot the results in per-unit values.
sim = model.Simulation(mdl, ctrl)
res = sim.simulate(t_stop=1.6)
utils.plot(res, base)
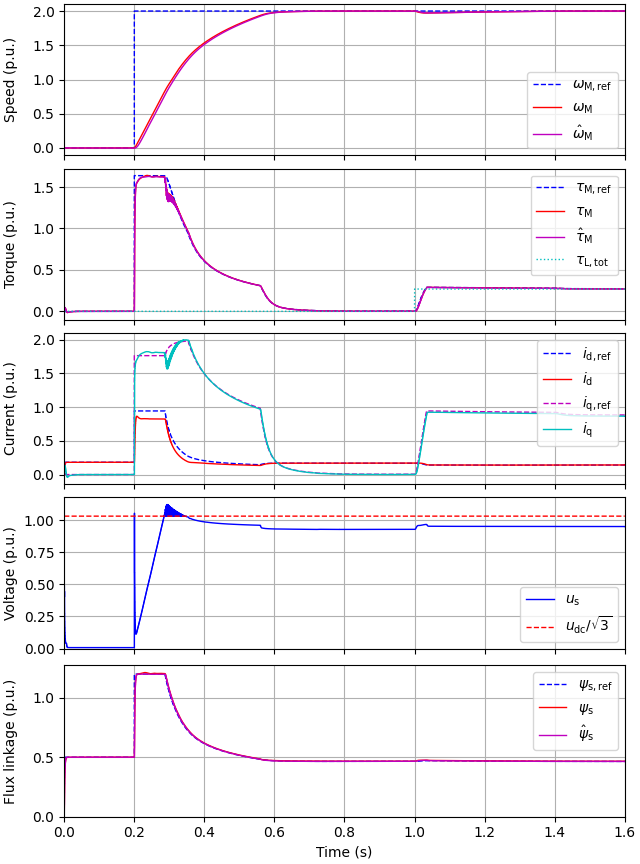
Total running time of the script: (0 minutes 22.242 seconds)